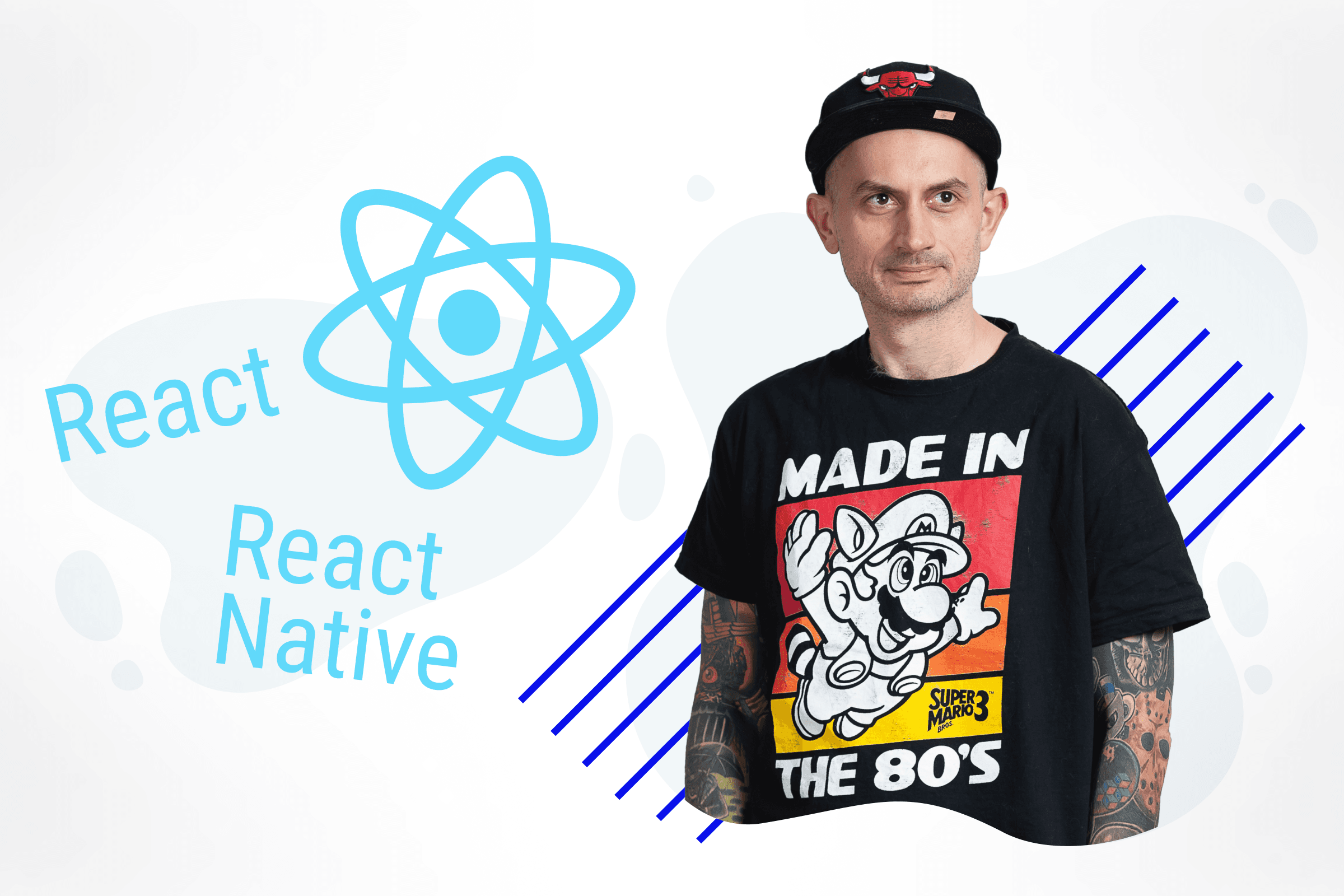
Why do you need automation?
When working on a new feature, improvement, or bug fix, it is crucial to ensure that all changes adhere to the rules specified by the linting tools, such as ESLint and/or prettier . Similarly, it is essential to run tests whenever changes are made to guarantee that there are no regressions.
With a simple project setup utilizing ESLint for linting, and jest and cypress for testing, at some point, you will need to execute the following command:
npx eslint . # linting
npx tsc # (only if using typescript) check types
npx jest # run jest unit tests
npx cypress run # run e2e tests with cypress
This is a lot to remember and as the project grows there can be more commands to run. It can become annoying and repetitive really fast to run all of these commands by hand. Moreover, forgetting to run some of them can cause production builds to fail or introduce bugs that could’ve been easily avoided by executing these commands locally.
It can become quite challenging to remember and manage all of these commands, especially as the project grows. The list of commands can quickly become overwhelming and repetitive to execute manually. Additionally, forgetting to run some of these commands can lead to production build failures or introduce bugs that could have been easily avoided by running these commands locally.
Fortunately, there are tools available that can assist you in configuring all the necessary commands to run automatically whenever you need them, relieving you of the need to think about them ever again.
Knock-out repetitiveness with Lefthook!
Lefthook is an incredibly useful, lightweight, and easy-to-configure tool that automates various aspects of your workflow, including code formatting, test execution, and more!
To begin, you'll need to install Lefthook in your project.
pnpm add -D lefthook
# or yarn
yarn add -D lefthook
# or npm
npm i -D lefthook
Configure it with ease
After the installation is complete, you can begin configuring Lefthook by creating a lefthook.yml
file in the project's root directory.
# lefthook.yml
pre-commit:
commands:
linting:
run: npx eslint .
typ-checking:
run: npx tsc --pretty
unit-testing:
run: npx jest
end-2-end-testing:
run: yarn dev & npx cypress run
So clean and simple! Now, whenever you commit changes, Lefthook will automatically execute all of these commands. Whenever you commit changes, you should expect to see something similar to this:
npx lefthook install
Built-in features
However, this script can be further improved. For example, there is no need to lint the entire project every time you commit changes. Ideally, linting should only be performed on the staged files.
Moreover, it would be beneficial if the script could also handle fixing any auto-fixable warnings and errors automatically.
Lefthook has you covered with built-in lists of files that can be used for that exact purpose for example {staged_files}
.
Updated configuration will look like this:
# lefthook.yml
pre-commit:
commands:
linting:
glob: “/*.{ts,tsx,js,jsx}”
run: npx eslint {staged_files} --fix && git add {staged_files}
stage_fixed: true
typ-checking:
run: npx tsc –-pretty
unit-testing:
run: npx jest
end-2-end-testing:
run: yarn dev & npx cypress run
-
staged_fixed: true
makes sure that any file that was changed by ESLint auto-fixing will be re-added to staged files. -
glob: “/*.{ts,tsx,js,jsx}”
makes sure that ESLint is run only against javascript / typescript files within all the staged files.
Running concurrently
Running each command individually can be time-consuming, particularly when there are numerous commands involved. To expedite the process, Lefthook can execute all the commands in parallel by adding a single line to the configuration file, as demonstrated below:
# lefthook.yml
pre-commit:
parallel: true
commands:
linting:
glob: “/*.{ts,tsx,js,jsx}”
run: npx eslint {staged_files} --fix
stage_fixed: true
typ-checking:
run: npx tsc –-pretty
unit-testing:
run: npx jest
end-2-end-testing:
run: yarn dev & npx cypress run
-
parallel: true
will run all the commands concurrently, which should make the whole process a little bit faster.
What else can be automated?
Another command that can be automated is the installation of packages whenever you merge changes into your working branch. Other developers may have added different packages at any given time, so it can be advantageous to install them automatically before starting your work.
How to achieve this in Lefthook:
#lefthook.yml
post-merge:
commands:
install-deps:
run: pnpm install
Important to note: post-merge
hook will not run if any conflicts were detected!
Whole lefthook.yml file should like like this:
post-merge:
commands:
install-deps:
run: pnpm install
pre-commit:
commands:
linting:
glob: "/*.{ts,tsx}"
run: npx eslint {staged_files} --fix
stage_fixed: true,
type-checking:
run: npx tsc --pretty
unit-testing:
run: npx jest
end-2-end-testing:
run: "yarn dev & npx cypress run"
Conclusion
Lefthook is a very powerful tool that can help with automating repetitive processes, thus improving the overall developer experience by allowing your team to focus on delivering code that will be linted and tested automatically.
It is very easy to configure in new and existing projects, so I highly recommend trying it out on your own!