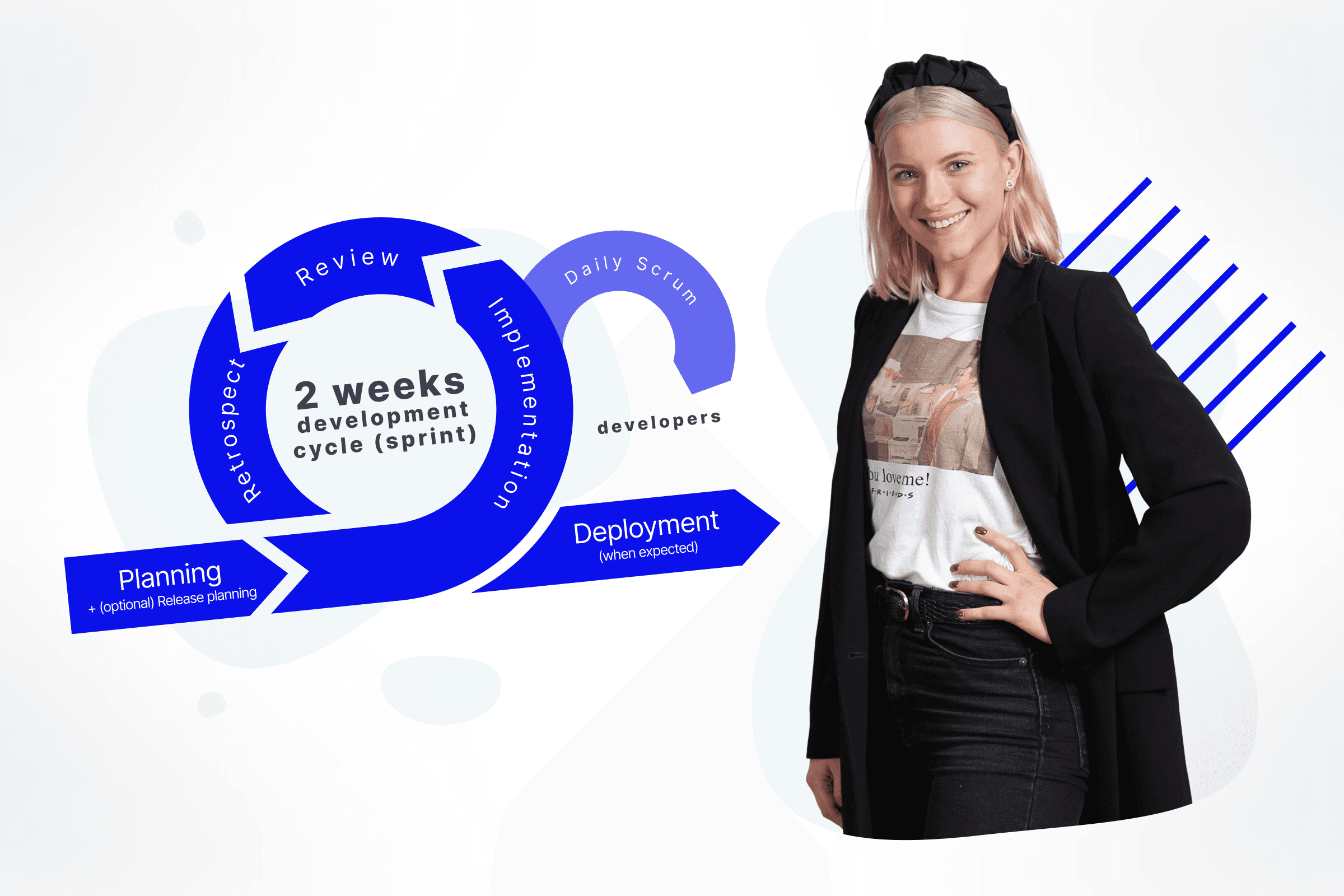
Prettier is like your very experienced colleague who constantly whispers advice about your code consistency and readability. Who wouldn’t want one?
Unfortunately, very experienced developers are often busy, and that’s why Prettier was created. In essence, it’s a tool that helps developers implement best coding practices automatically.
Here is a concise Prettier guide including key benefits, React and Typescript code examples, and comments from our developers.
Key benefits
-
Opinionated Formatting: Prettier has a set of default formatting rules based on community best practices, making it easy to format your code without spending time on style debates.
-
Multiple Language Support: Prettier supports various programming languages such as JavaScript, TypeScript, CSS, HTML, and more.
-
Customizable Rules: Prettier allows you to customize the formatting rules to fit your project's specific needs, giving you more control over your code formatting.
-
Easy Integration: Prettier can be integrated into your development workflow with ease, enabling you to automate your code formatting and improve your productivity.
-
Improved Readability: By formatting your code consistently and following best practices, Prettier can make your code more readable and easier to understand.
-
Reduced Maintenance Time: Prettier can save you time on code maintenance, as it can automatically format your code as you write it, reducing the need for manual formatting.
-
Reduced Human Error: By automating the code formatting process, Prettier can reduce the chance of human error and ensure that your code is always formatted correctly.
How does Prettier work?
Prettier parses your code into an abstract syntax tree (AST) using a parser specific to the programming language you are using.
-
01.
Applies a set of formatting rules to the AST, modifying it to conform to the formatting rules. These rules are based on community best practices and are designed to ensure consistency and readability in your code.
-
02.
Generates formatted code based on the modified AST, producing a new code file that conforms to the formatting rules.
Prettier also provides options to customize the formatting rules, allowing you to tailor the formatting to your project's specific needs. Once configured, you can integrate Prettier into your development workflow using various tools such as code editors or build scripts. This way, you can automate your code formatting and maintain a consistent code style across your team.
How to install Prettier?
To configure Prettier, you can create a configuration file in your project's root directory. Here is the step-by-step guide:
-
01.
Install Prettier: You can install Prettier either globally or locally to your project using a package manager like npm or yarn. For example, to install Prettier globally, you can run the following command in your terminal:
npm install -g prettier
-
01.
Create a configuration file: Create a
.prettierrc
orprettier.config.js
file in your project's root directory. -
02.
Specify formatting rules: In your configuration file, you can specify the formatting rules you want Prettier to apply. You can find a list of available options in the Prettier documentation. Here's an example
.prettierrc
file that sets the maximum line length to 80 characters and uses single quotes for string literals:
{
"printWidth": 80,
"singleQuote": true
}
How does Prettier work with JavaScript and Typescript?
Here are some examples of how Prettier works with JavaScript and TypeScript:
-
01.
Formatting JSX
Before:
import "./App.css";
import Scheduler from "./components/Scheduler";
function App() {
return (
<div className="App">
<Scheduler />
</div>
);
}
export default App;
After:
import "./App.css";
import Scheduler from "./components/Scheduler";
function App() {
return (
<div className="App"><Scheduler /></div>
);
}
export default App;
-
01.
Formatting conditionals
Before:
const Component = () => {
return (
<>
{ hasName
&&
<h1>John Doe</h1>
}
{!!hasName
&& <h1>Unknown name</h1>}
</>
);
};
export default Component;
After:
const Component = () => {
return (
<>
{hasName && <h1>John Doe</h1>}
{!!hasName && <h1>Unknown name</h1>}
</>
);
};
export default Component;
-
01.
Formatting TypeScript in a Functional Component
Before:
import React from 'react';
interface Props { name: string; age:
number; }
const Component: React.FC<Props>= ({name
,age}) => {
return (
<>
<h1>{name}</h1><p>{`Age: ${age}`}</p>
</>
);
};
export default Component;
After:
import React from "react";
interface Props {
name: string;
age: number;
}
const Component: React.FC<Props> = ({
name, age }) => {
return (
<>
<h1>{name}</h1>
<p>{`Age: ${age}`}</p>
</>
);
};
export default Component;
What are alternative and complementary tools?
-
01.
ESLint : ESLint is a popular static analysis tool for identifying problematic patterns found in JavaScript code. It has built-in rules and plugins for code formatting and styling.
-
02.
JSHint : JSHint is a community-driven tool that helps detect errors and potential problems in JavaScript code. It has built-in options for enforcing code style and formatting.
-
03.
StandardJS : StandardJS is a style guide and linter for JavaScript code. It has a built-in code formatter and can be integrated into various editors and IDEs.
-
04.
Beautify : Beautify is a code formatter that can be used with various programming languages, including JavaScript. It has plugins for popular editors and IDEs like VS Code, Atom, and Sublime Text.
-
05.
CodeMirror : CodeMirror is a versatile text editor for the web that can be used for formatting and styling code. It has built-in features for code highlighting, indentation, and autocomplete.
-
06.
JS Beautifier : JS Beautifier is a simple tool that can be used for formatting and beautifying JavaScript code. It has options for configuring the code style and formatting rules.
-
07.
Black: Black is a Python-based code formatter that can be used for formatting code in various programming languages, including JavaScript. It has options for configuring the code style and formatting rules.
Summary
Prettier is a code formatting tool that automatically implements best coding practices, and improves code consistency, and readability.
It has default formatting rules based on community best practices, supports multiple programming languages, is customizable, and can be easily integrated into a development workflow. Prettier works by parsing code into an AST, applying formatting rules, and generating new formatted code. Developers can install Prettier by creating a configuration file with specified formatting rules. Prettier works - among others - JavaScript and TypeScript.
I encourage you to try out Prettier and other similar tools.
If you have any questions or are interested in our services, do not hesitate to reach out to us.